ElasticSearch-high-level-client 是 java 操作 ElasticSearch 的一个客户端 API
1. 引入依赖
1 2 3 4 5 6 7 8 9 10
| <properties> <elasticsearch.version>7.4.2</elasticsearch.version> </properties> <dependencies> <dependency> <groupId>org.elasticsearch.client</groupId> <artifactId>elasticsearch-rest-high-level-client</artifactId> <version>7.4.2</version> </dependency> </dependencies>
|
2. 测试保存数据
下面测试测试索引保存数据到 ES 中,具体步骤如下,可以参考官网进行:Java High Level REST Client
1)构建 RestHighLevelClient ,该类用于与 ES 做交互,我们将其创建加入 IOC 容器之中,如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| @Configuration public class GulimallElasticSearchConfig {
private static final String HOST_IP = "192.168.160.129"; private static final int PORT = 9200; private static final String SCHEME = "http";
@Bean RestHighLevelClient client() { RestClientBuilder builder = RestClient.builder(new HttpHost(HOST_IP, PORT, SCHEME)); return new RestHighLevelClient(builder); } }
|
2)请求统一定制,便于对 ES 的请求进行统一处理,比如设置请求头和响应等;下面仅进行简单的使用,还是同一个类:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| @Configuration public class GulimallElasticSearchConfig {
private static final String HOST_IP = "192.168.160.129"; private static final int PORT = 9200; private static final String SCHEME = "http"; public static final RequestOptions COMMON_OPTIONS;
static { RequestOptions.Builder builder = RequestOptions.DEFAULT.toBuilder(); COMMON_OPTIONS = builder.build(); }
@Bean RestHighLevelClient client() { RestClientBuilder builder = RestClient.builder(new HttpHost(HOST_IP, PORT, SCHEME)); return new RestHighLevelClient(builder); } }
|
3)创建测试类,具体如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| @RunWith(SpringRunner.class) @SpringBootTest @Slf4j public class GulimallSearchApplicationTests {
@Autowired private RestHighLevelClient client;
@Data @ToString class Product { private String spuName; private Long id; }
@Test public void test1() throws IOException { Product product = new Product(); product.setSpuName("华为"); product.setId(10L); String productJsonStr = JSON.toJSONString(product); IndexRequest request = new IndexRequest("product"); request.id("1"); request.source(productJsonStr, XContentType.JSON); IndexResponse indexResponse = client.index(request, GulimallElasticSearchConfig.COMMON_OPTIONS); log.info("响应信息:{}", indexResponse.toString()); }
}
|
4)运行成功后,可以在 kibana 操作页面查询索引进 ES 的数据:
1 2 3 4 5 6
| GET product/_search { "query": { "match_all": {} } }
|
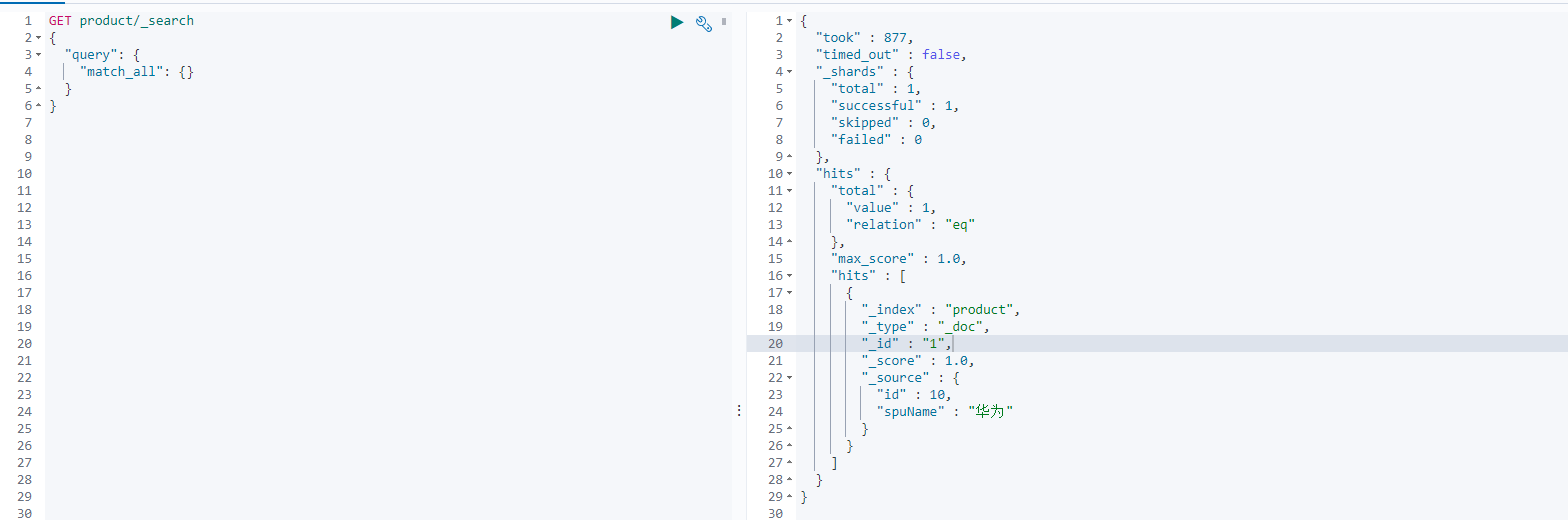
更多操作,可以参照官方文档~